C Programming of the PACSystems Controllers includes a main function named GEFMain. Input and Output parameters can be passed to the GEFMain function when called from Ladder, ST, ILS, or Function Block Diagram. Parameters are passed to C Blocks by address, therefore your C code could operate using pointer manipulation. However, this is not a recommended best practice as it is error prone and may result in crashing the PLC if a pointer value is not correct and causes overrun of the variable storage memory. As a best practice one should define the GEFMain input/output parameters as an array of pointers inside the C program, thus allowing use of the array subscript within the C program. One such example is seen below:
//******************** Technical Support April 2022 ********************
// This program is the source code used to create a PLC C Executable Program Block for the PACSystems products,
// when compiled using the PACSystems C Toolkit GNU compiler command:
// compileCPACRX <file name>
// Where <file name> is the C Source file name with no file extension, as documented in GFK-2259.
// This example includes the standard header files needed for input/output and the custom PLC C Functions/Macros
// and Data Types available in the PACSystems Controllers as a generally accepted starting point.
//***************************************************************************************************************
// Include necessary header files
#include <stdio.h>
#include <PACRXPlc.h>
#include <ctkPLCUtil.h>
// Define the function GEFMain as needed in all cases. In this case GEFMain is a Funtion that will return a
// 32 bit PLC Type Integer and requires two input parameter pointers X1, and Y1, where X1 is an Input
// parameter and Y1 is an Output parameter pointer from the PLC Calling Logic. Note that ALL C Block
// parameters are passed in and out as pointers.
//**********************
T_INT32 GefMain (X1, Y1)
// Declare GEFMain Input Parameter Data Types
T_REAL32 X1[4]; //X1 - as an array of 4 PLC Type T_REAL32
T_REAL32 Y1[4]; //Y1 - as an array of 4 PLC T_REAL32
// Note that the above could optionally be combined into a single line as below:
// T_INT32 GefMain (T_INT32 X1[4], T_INT32 Y1[4])
//**********************
{
//Move Input Array X1[] to Output Array Y1[], 1 element at a time
Y1[0]=X1[3];
Y1[1]=X1[2];
Y1[2]=X1[1];
Y1[3]=X1[0];
// Return the execution ok status to drive the PLC function OK output high upon execution completion
return(GEF_EXECUTION_OK);
}
See below screen shot for details about the ladder call and matching parameter definitions. Notice that the PLC Ladder Variable Name does not need to match the C variable names as the C variable simply inherits the PLC Ladder variable address. Also note that the Input and Output parameter variables are defined as Real Arrays of Length 4 to match the C block definition inside GEFMain. This is important as a mismatch may also crash the PLC due to the C reaching beyond the defined Ladder Variable Length.
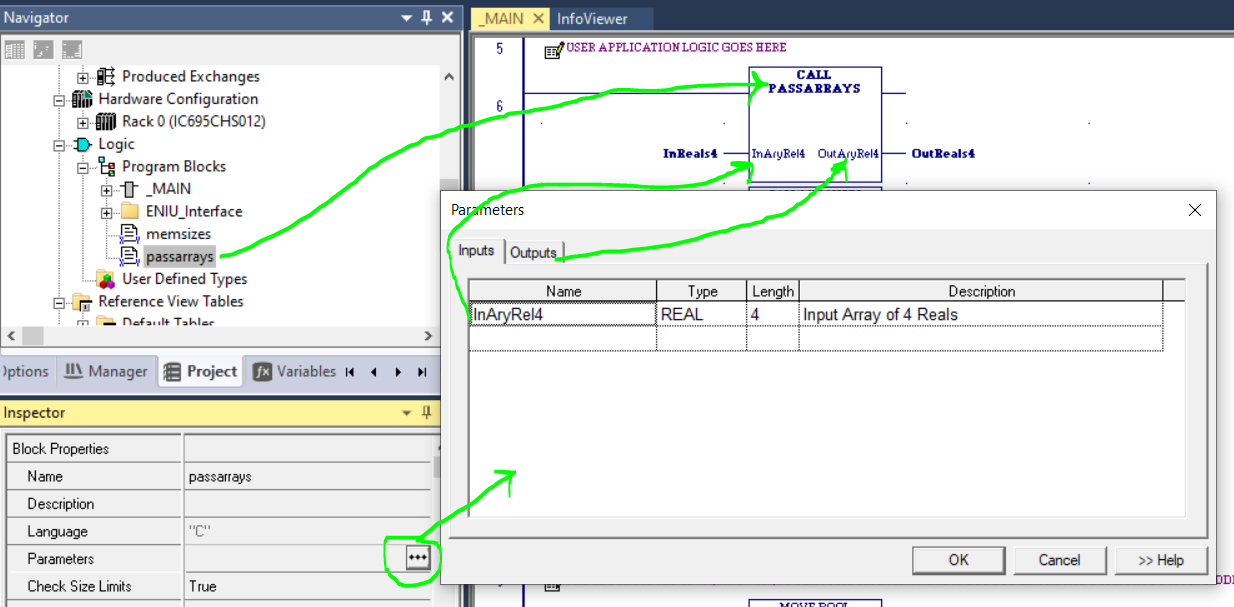
There are many small examples in the PACSystems C Toolkit manual, GFK-2259.
See PACSystems C Toolkit - Landing Page for Documentation, the various Compilers, informational articles, and programming examples.
PACSystems C Toolkit - Landing Page
//******************** Technical Support April 2022 ********************
// This program is the source code used to create a PLC C Executable Program Block for the PACSystems products,
// when compiled using the PACSystems C Toolkit GNU compiler command:
// compileCPACRX <file name>
// Where <file name> is the C Source file name with no file extension, as documented in GFK-2259.
// This example includes the standard header files needed for input/output and the custom PLC C Functions/Macros
// and Data Types available in the PACSystems Controllers as a generally accepted starting point.
//***************************************************************************************************************
// Include necessary header files
#include <stdio.h>
#include <PACRXPlc.h>
#include <ctkPLCUtil.h>
// Define the function GEFMain as needed in all cases. In this case GEFMain is a Funtion that will return a
// 32 bit PLC Type Integer and requires two input parameter pointers X1, and Y1, where X1 is an Input
// parameter and Y1 is an Output parameter pointer from the PLC Calling Logic. Note that ALL C Block
// parameters are passed in and out as pointers.
//**********************
T_INT32 GefMain (X1, Y1)
// Declare GEFMain Input Parameter Data Types
T_REAL32 X1[4]; //X1 - as an array of 4 PLC Type T_REAL32
T_REAL32 Y1[4]; //Y1 - as an array of 4 PLC T_REAL32
// Note that the above could optionally be combined into a single line as below:
// T_INT32 GefMain (T_INT32 X1[4], T_INT32 Y1[4])
//**********************
{
//Move Input Array X1[] to Output Array Y1[], 1 element at a time
Y1[0]=X1[3];
Y1[1]=X1[2];
Y1[2]=X1[1];
Y1[3]=X1[0];
// Return the execution ok status to drive the PLC function OK output high upon execution completion
return(GEF_EXECUTION_OK);
}
See below screen shot for details about the ladder call and matching parameter definitions. Notice that the PLC Ladder Variable Name does not need to match the C variable names as the C variable simply inherits the PLC Ladder variable address. Also note that the Input and Output parameter variables are defined as Real Arrays of Length 4 to match the C block definition inside GEFMain. This is important as a mismatch may also crash the PLC due to the C reaching beyond the defined Ladder Variable Length.
There are many small examples in the PACSystems C Toolkit manual, GFK-2259.
See PACSystems C Toolkit - Landing Page for Documentation, the various Compilers, informational articles, and programming examples.
PACSystems C Toolkit - Landing Page